Book Query Application Project Using Python [2023]
![Book Query Application Project Using Python [2023]](https://assets-global.website-files.com/60d04c4106d835bf99aa4c35/63abe4c90281d20ac3b3da2f_Book%20Query%20Application%20Project%20Using%20Python%20%5B2023%5D.jpg)
In this Book Query Application Session Using Python, "Aneeq Dholakia," co-founder of Edyst, teaches attendees about
1. Accessing details of any book.
2. Finding the details of the author of multiple books.
3. Finding commonality between 2 authors based on publishing dates.
We will have two milestones for this Book Query Application session:
1. We will take the book's name as input and publish the Author's name and book publishing year as output.
2. We will take the names of two books as input and publish the subjects common in both. We will ensure that we print the output in alphabetical order of subjects. For simplicity we will be using these two books:
- Lord of the flies
- Animal Farm
We will return the common subjects of these two books using the information on the command subjects of these two books mentioned at the open library website.
Here is the website URL: https://openlibrary.org/developers/api
We will use the above mentioned URL for the data.
Below is the entire program flow:
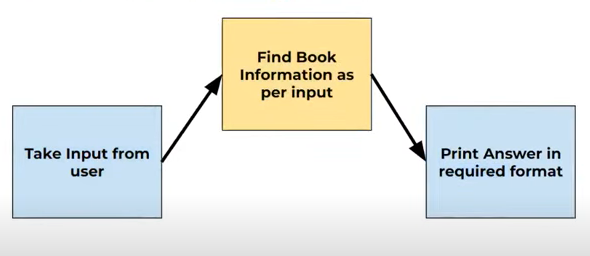
Also,we will be using 3 simple concepts for creating the Book Query Application in Python:
1. APIs
2. JSON
3. Data Structures
Below are the details of these concepts:
Note: You can skip the details of the below concepts if you are familiar with them and proceed towards the main section.
1. Application Programming Interface (APIs)
An API refers to a set of rules and protocols that define how different software systems can interact with each other. APIs allow different software systems to communicate with each other and share data and functionality.
APIs often use HTTP as the underlying protocol for communication, and they usually expose a set of URLs (endpoints) that can be accessed using HTTP methods such as GET, POST, PUT, and DELETE. Each endpoint typically represents a specific resource or collection of resources, and the HTTP method specifies the type of operation to be performed on the resource(s).
APIs are used in many different contexts, including web development, mobile app development, and data integration. For example, a web developer might use an API to retrieve data from a database and display it on a website, or a mobile app developer might use an API to access a third-party service such as a payment gateway or a social media platform.
2. JavaScript Object Notation (JSON)
JSON is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript programming language and is used to represent data in a structured way, like in a dictionary or a list.
It is used to transmit data between a server and a web application, or between two servers. It is also commonly used for storing data in a NoSQL database, such as MongoDB.
3. Data Structures
It refers to the ways of organizing and storing data in a computer so that it can be accessed and modified efficiently. Some common examples of data structures include:
Arrays: An array is a contiguous block of memory that stores a fixed-size sequence of elements of the same type. Arrays are indexed by integer values and are efficient for storing and accessing data sequentially.
Linked lists: A linked list is a linear data structure that consists of a sequence of nodes, where each node stores a reference to the next node in the sequence. Linked lists are useful for inserting and deleting elements at arbitrary positions, but they are not as efficient as arrays for accessing elements by index.
Stack: A stack is a last-in, first-out (LIFO) data structure that supports two basic operations: push, which adds an element to the top of the stack, and pop, which removes the element from the top of the stack. Stacks are often used for temporary storage, such as for function calls in a recursive algorithm.
Queue: A queue is a first-in, first-out (FIFO) data structure that supports two basic operations: enqueue, which adds an element to the back of the queue, and dequeue, which removes the element from the front of the queue. Queues are often used for storing data that needs to be processed in a specific order.
Tree: A tree is a data structure that consists of a set of nodes connected by edges. Each node has zero or more child nodes, and the top node is called the root. Trees are often used to represent hierarchical structures, such as the file system on a computer.
Graph: A graph is a data structure that consists of a set of vertices (or nodes) and a set of edges that connect them. Graphs can be used to represent networks or relationships between objects.
If you still need more information on the 3 concepts, please check our FREE resource module here https://edyst.com/events/books-query-application-free-project-with-certificate
Note: You have to login to view the FREE module.
Practical Session Milestone I
We will begin by opening a tool https://colab.research.google.com/
We use colab.research.google.com to open Jupyter notebooks hosted on Google Cloud. Using it we can create and run Jupyter notebooks on Google's servers without having to install any software on our own computer.
To open a Python notebook follow these steps:
- Go to colab.research.google.com in your web browser and sign in with your Google account.
- Click on the "New Notebook" button.
- A new notebook will be created and opened in a new tab.
That's it!
We can now write and run Python code in our Jupyter notebook.
Next we will go to https://openlibrary.org/developers/api to understand the shape of the data.
- Visit https://openlibrary.org/developers/api
- Click on Search query
- Click on http://openlibrary.org/search.json?title=the+lord+of+the+rings under URL format
- The above link should show how you know the data you need to answer your query. At this stage you should copy this URL and return back to your notebook.
In your notebook start coding now,
import requests
resp = requests.get("http://openlibrary.org/search.json?title=the+lord+of+the+rings")
This code will import the requests library, and then use it to send a GET request to the Open Library Search API with the parameter title set to "The Lord of the Rings". The API will return a JSON object containing the search results which will be stored in resp.
Because our objective is to get data about the books with the title “To kill a mockingbird” replace the title of the book in the above code, below is how it should look like
import requests
resp = requests.get("http://openlibrary.org/search.json?title=to+kill+a+mockingbird")
Run your request now. If the request is successful (i.e., the status code 200 will show up, if not status code 404 will show up).
Next, you should store the data into a dictionary in python, you can do this using the below command
info = resp.json()
This command will use resp which is a Response object that contains a JSON object as its content and apply the .json() method to parse the JSON content and return it as a Python dictionary
Now in order to get author name and publishing year you should check info.keys(), below are the commands you should run
info.keys()
This command prints the keys of the info dictionary. This will show you the different fields that are available in the JSON object.
info['docs'][0].keys()
The command prints the keys of the first element in the docs array, which is a list of dictionaries containing information about the books that were found in the search.
print(info['docs'][0]['author_name'][0])
print(info['docs'][0]['first_publish_year'])
The above two commands will print the author_name and first_publish_year fields of the first book in the search results. The author_name field is a list of strings, so the first element is accessed using the [0] index.
Now we will make the space for input as the name of the book, so modify your command like this:
book_name = input()
This command prompts the user to enter a book name and stores the user's input in the book_name variable.
query_book = book_name.lower()
This command converts the book_name variable to lowercase and stores it in the query_book variable.
query_book = query_book.replace(' ', '+')
This command replaces all spaces in the query_book string with plus signs and stores the modified string back in the query_book variable.
print(query_book)
This command prints the modified query_book string.
resp = requests.get(f"http://openlibrary.org/search.json?title={query_book}")
This command sends a GET request to the Open Library Search API with the title parameter set to the user-specified book name, with spaces replaced by plus signs. The API will return a JSON object containing the search results for books with the specified title. The requests.get() function returns a Response object, which contains various attributes such as the status code of the response, the headers, and the content of the response.
info = resp.json()
The command parses the JSON content of the Response object and returns it as a Python dictionary. The info variable will now contain a dictionary that represents the JSON object returned by the Open Library Search API.
author_name = info['docs'][0]['author_name'][0]
publishing_year = info['docs'][0]['first_publish_year']
The above two commands retrieve the author_name and first_publish_year fields of the first book in the search results and store them in the author_name and publishing_year variables, respectively. The author_name field is a list of strings, so the first element is accessed using the [0] index.
print(f"Author of '{book_name}' is {author_name}. It was first published in {publishing_year}")
The command prints a string containing the book name, author, and publishing year of the first book in the search results. The f at the beginning of the string indicates that the string is a "f-string", which allows you to embed expressions inside curly braces that will be evaluated and formatted into the final string.
Overall, the above commands allows the user to enter a book name, search for books with the specified title using the Open Library Search API, and print the author and publishing year of the first book in the search results. This as our milestone #1 now, we will work on milestone #2.
Practical Session Milestone II
In your new notebook start coding now,
import requests
The command imports the requests module, which is a library for making HTTP requests in Python
def get_subject(book_name):
The command takes a book name as input.
query_book = book_name.lower()
query_book = query_book.replace(' ', '+')
The above two commands convert the book name to lowercase and replace any spaces with plus signs (+). This is done to ensure that the book name can be used as a query parameter in the URL for the OpenLibrary API.
resp = requests.get(f"http://openlibrary.org/search.json?title={query_book}")
It uses the requests.get function to make a GET request to the OpenLibrary API with the book name as a query parameter. The f before the URL string indicates that it is an f-string, which allows the book name to be easily inserted into the string using curly braces {}.
info = resp.json()
It retrieves the JSON-formatted response from the API and parses it into a Python dictionary using the json method.
subjects = info['docs'][0]['subject']
It retrieves the subject information for the book from the dictionary using the keys 'docs' and 'subject', and assigns it to the subject's variable.
return subjects
It returns the subjects variable.
book1 = input()
book2 = input()
The above two commands ask the user to input the names of two books and store them in the variables book1 and book2.
subj1 = get_subject(book1)
subj2 = get_subject(book2)
This command uses the get_subject function on each book name and stores the returned subject information in the variables subj1 and subj2.
s1 = set(subj1)
s2 = set(subj2)
The set function is then called on subj1 and subj2 to create sets of unique subjects for each book. The sets are stored in the variables s1 and s2.
common = s1 & s2
This command uses & operator to find the intersection of the two sets, s1 and s2, which represents the common subjects between the two books. The result of the intersection is assigned to the common variable.
common = list(common)
This command uses a list function and is then called on common to convert it from a set object to a list object.
common.sort()
This command applied the sort method on the common list to sort it in alphabetical order.
print('Common subjects are:', end=' ')
The command is used to print a string and a list of the common subjects. The end parameter is set to a space character to ensure that the subjects are printed with a space between them, rather than on separate lines.
for subject in common:
print(subject, end= ' ')
The for loop iterates over the elements in the common list, and the print function is used to print each element with a space between them. The end parameter is set to a space character to ensure that the subjects are printed with a space between them, rather than on separate lines.
print()
Finally, a new line is printed using the print function with no arguments. This causes the loop to print all the elements of the common list on the same line, separated by spaces.